The Top Ways for Smart Contract Developers to Use Chainlink
With massive DeFi adoption, more attention than ever is being paid to blockchain oracle solutions and how to best leverage them to access high-quality data feeds in a secure manner. For smart contracts, a reliable mechanism for interacting with data outside of their underlying blockchains is critical to providing real-world value. At Chainlink, we offer a suite of oracle solutions for smart contract developers that facilitate a multitude of different interactions between their on-chain applications and off-chain data.
Core Functionality for Smart Contract Developers
- Price Feeds – Accurate, decentralized price data with broad market coverage
- Access Any API – POST, GET and more with any API
- Chainlink VRF – Generate provably random numbers
Chainlink Price Feeds
Price Feeds are on-chain resources containing price data that are aggregated from multiple independent, high quality data sources and multiple independent, Sybil Resistant node operators on the Chainlink Network. They’re fast, reliable, decentralized, executed in a single transaction, and are the easiest way to obtain asset price data from oracles. Chainlink Price Feeds are the widely used oracles in the DeFi space, with several large players like Synthetix and AAVE trusting them for accurate data to price synthetic assets or determine the value of loans and collateral.
If you’re writing smart contracts that require accurate, decentralized crypto prices, Price Feeds are the perfect tool. Chainlink Price feeds are so easy to use they can be implemented with just a few lines of solidity code, with a single function call to retrieve the desired price data.
pragma solidity ^0.6.7; import "@chainlink/contracts/src/v0.6/interfaces/AggregatorV3Interface.sol"; contract PriceConsumerV3 { AggregatorV3Interface internal priceFeed; constructor() public { priceFeed = AggregatorV3Interface(feedAddress); } function getLatestPrice() public view returns (int) { ( uint80 roundID, int price, uint startedAt, uint timeStamp, uint80 answeredInRound ) = priceFeed.latestRoundData(); return price; } }
Access Any API
If you’re writing contracts that need any off-chain data other than the default aggregated price data, Chainlink provides Any API functionality. Whether your contracts need sports results, weather forecasts, flight times, or any other data available through an API, Chainlink enables your contract to consume it and also output results back to the external world. The ability to bring any API into smart contracts is driving several valuable use cases live in-production today, such as Arbol’s farming coverage dApp. Arbol uses decentralized weather data obtained through Chainlink to power their parametric weather coverage product, where contract payouts are automatically and trustlessly enforced by conditional data such whether a certain amount of rainfall occurred at a specific geographic location over a defined amount of time. This eliminates middlemen overhead and potential disputes over mismatched payout conditions.
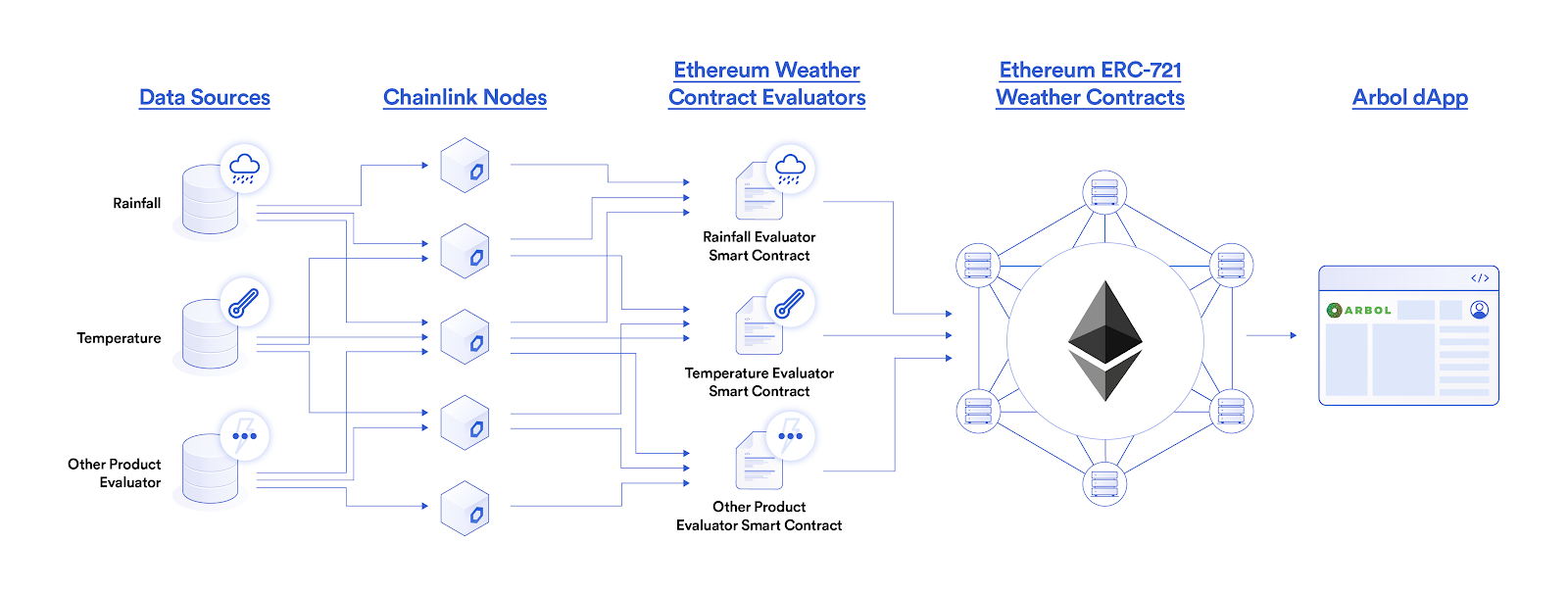
This request and receive functionality is the primary framework by which smart contracts can interact with Chainlink’s network of decentralized oracles. All your smart contract needs to do is extend our smart contract library, build a request, and implement a callback function to receive the response. Chainlink External Adapters can also further empower your smart contract by enabling any kind of off-chain data access and manipulation you can think up.
This powerful tool opens up unlimited possibilities for smart contracts to represent assets, and act upon real-world events. See our article on connecting a smart contract to any API to learn how your contracts can easily interact with off-chain data through Chainlink.
Chainlink VRF
Randomness may seem simple, but in reality, it’s difficult to generate unbiasable randomness in a decentralized network. Using “randomness” from on-chain data, such as blockhashes, is suboptimal because it can be tampered with by miners. For example, a miner deciding to post or not post a block based on their position in a lottery and how the blockhash will affect them. We created Chainlink VRF to fix these issues and provide verifiable randomness that can be used securely inside smart contracts.
With Chainlink VRF, you can both generate a random number off-chain where it’s not susceptible to on-chain concerns, and then cryptographically prove that it has not been tampered with. This is a huge leap for decentralized randomness because developers and users can now cryptographically verify that the generation of the “random” number has not been manipulated by the source. With this functionality, many new smart contract dApps are now possible. The multi-billion dollar market of in-game item generation is now expanding into blockchain games which, with VRF, can generate NFT rewards based on true, verifiable randomness.
How this works is a seed value is first provided by the requesting contract to the Chainlink oracles. Then the oracles generate the random value using the provided seed in conjunction with their secret key and commit that to the VRF verification contract along with their cryptographic proof. Since the VRF contract knows the oracles’ public keys, which correspond to their private keys and the seed value, it can then verify the proofs. If any node’s answer was manipulated, its proof would not match what the VRF contract calculates and it would be rejected as tampered data. Similar to how you can quickly verify a file download’s contents with its MD5 hash, the VRF contract is verifying the random numbers reported, but instead of a simple hash, asymmetric key pairs and cryptographic proofs are used.
So how can blockchain developers access this powerful new functionality? As with our aggregated price data, we’ve made it incredibly easy to integrate. The example code block below can be used as a standard template for contracts that wish to consume VRF data.
pragma solidity 0.6.6; import “@chainlink/contracts/src/v0.6/VRFConsumerBase.sol”; contract RandomNumberConsumer is VRFConsumerBase { bytes32 internal keyHash; uint256 internal fee; uint256 public randomResult; constructor() VRFConsumerBase( 0x2e184F4120eFc6BF4943E3822FA0e5c3829e2fbD, // VRF Coordinator 0x20fE562d797A42Dcb3399062AE9546cd06f63280 // LINK Token ) public { keyHash = 0x757844cd6652a5805e9adb8203134e10a26ef59f62b864ed6a8c054733a1dcb0; fee = 0.1 * 10 ** 18; // 0.1 LINK } /** * Requests randomness from a user-provided seed */ function getRandomNumber(uint256 userProvidedSeed) public returns (bytes32 requestId) { require(LINK.balanceOf(address(this)) > fee, "Not enough LINK - fill contract with faucet"); return requestRandomness(keyHash, fee, userProvidedSeed); } /**
Simply import VRF, construct your contract with the address and key hash of the VRF contract you are using, then provide two functions, getRandomNumber
, which sends the uint256 seed that the client generated as desired, and fulfillRandomness
, which is the callback function the VRF contract uses to return the verified random value. That’s it; import, constructor, two functions, and your contract is now verifiably random.
Hopefully this article has helped expand your knowledge of what you can build in-production today with Chainlink. Whether it’s price data to secure DeFi, any API to trigger a smart contract application, or provably fair randomness to seed in-game outcomes, Chainlink is there to enable it.
Start Building with Chainlink Today
If you learned something new here, why not try your skills at the Chainlink Hackathon? We are awarding over $40k of prizes and we highly recommend you take part.
If you’re reading this after the hackathon, be sure to join the community on Twitter, Discord, YouTube, or Reddit, and hashtag your repos with #chainlink and #ChainlinkEA.
If you’re developing a product that could benefit from Chainlink oracles or would like to assist in the open-source development of the Chainlink network, visit the developer documentation or join the technical discussion on Discord.