How to Build and Deploy an Avalanche Smart Contract
In this technical tutorial, we’ll walk through how to build and deploy a smart contract to the Avalanche Contract-Chain (C-Chain), as well as how to leverage Chainlink Data Feeds to create hybrid smart contracts on Avalanche that use off-chain market data.
With Chainlink Price Feeds now live on Avalanche mainnet, developers are able to easily leverage the Chainlink Network’s battle-tested, reliable price data at the full native speed and low cost of the Avalanche platform. Chainlink Price Feeds combine data from multiple liquidity sources and pre-aggregates through a decentralized network of Chainlink node operators to arrive at a single, highly accurate price with market-wide coverage.
Access to this data is critical to the growth of new chains, as there are many dApps that simply cannot exist without price or other external data. Avalanche is the latest example of a blockchain ecosystem seeing explosive growth following the deployment of Chainlink feeds, with TVL, transactions, and addresses all up since Chainlink integration, more developers than ever want to know how to build and deploy an Avalanche smart contract.
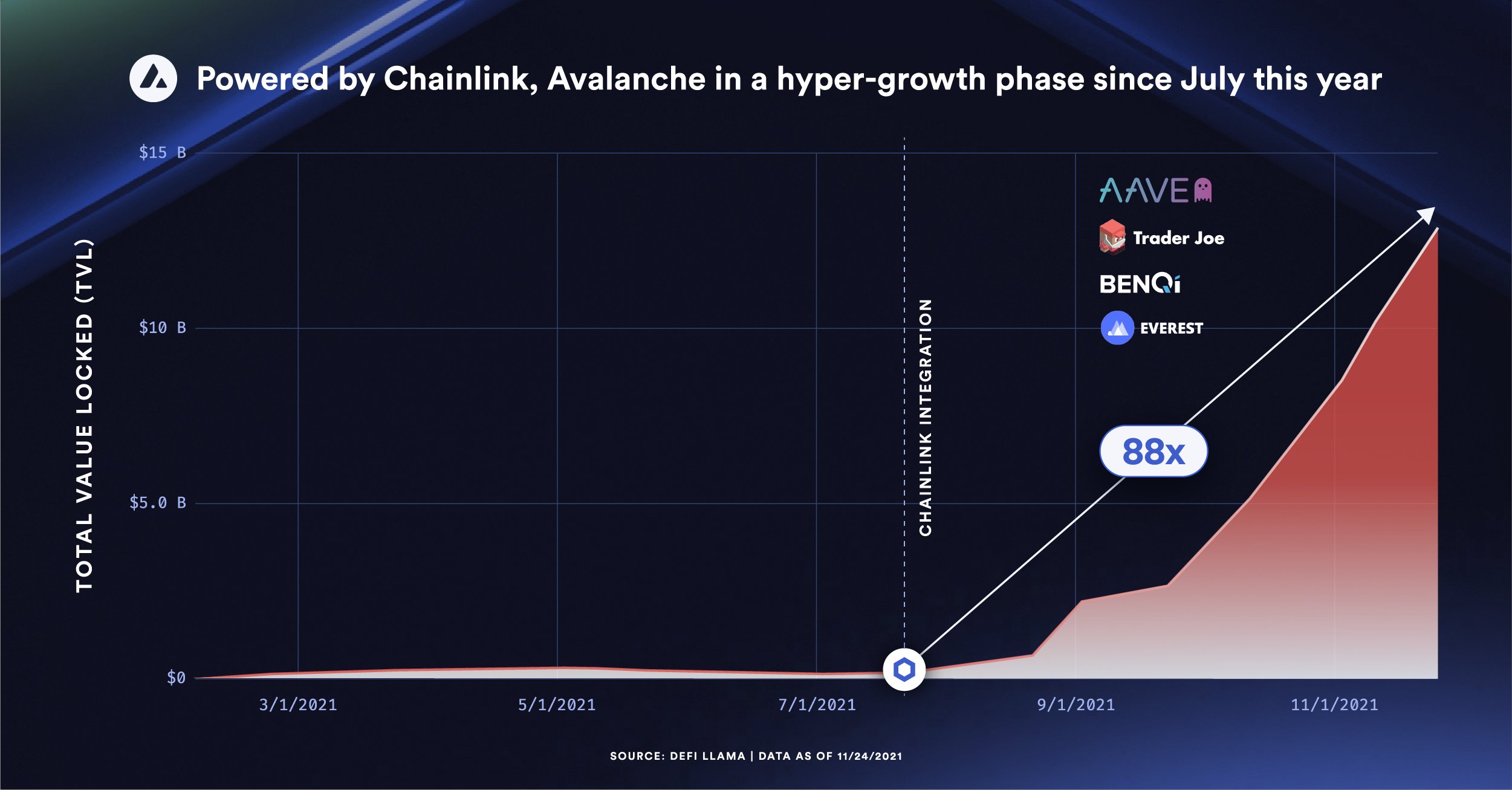
The integration of Chainlink Price Feeds and the recent launch of Avalanche Rush, one of the largest DeFi incentive programs in the world, make now the perfect time to learn how to deploy a dApp on Avalanche. In this tutorial, we’ll cover how to build and deploy a Solidity smart contract that retrieves Chainlink price data, which can be used to determine required loan collateral, exchange rates between tokens, reward rates for participants in dApps, and much more. Avalanche offers a high level of throughput, low-latency transaction finality, and a scalable, highly decentralized validator architecture thanks to its novel consensus algorithm. Additionally, since Avalanche is EVM-compatible, we can use all the standard Ethereum tooling. In this case, we’re building within Remix, the browser-based Solidity IDE.
You can follow along and deploy the code yourself using this Remix Gist.
// SPDX-License-Identifier: MIT pragma solidity 0.8; import "@chainlink/contracts/src/v0.8/interfaces/AggregatorV3Interface.sol"; contract AvaxLinkFeeds { AggregatorV3Interface internal priceFeed; /** * Network: Fuji * Aggregator: AVAX/USD * Address: 0x5498BB86BC934c8D34FDA08E81D444153d0D06aD * URL: https://docs.chain.link/docs/avalanche-price-feeds/ */ constructor() { priceFeed = AggregatorV3Interface(0x5498BB86BC934c8D34FDA08E81D444153d0D06aD); } /** * Returns the latest price */ function getLatestPrice() public view returns (int) { ( uint80 roundID, int price, uint startedAt, uint timeStamp, uint80 answeredInRound ) = priceFeed.latestRoundData(); return price; } }
Writing the Contract
We’ll start by importing the necessary Chainlink contract for price feeds, `AggregatorV3Interface.sol`, which contains the interface for retrieving data from existing pre-aggregated decentralized price feeds. To use this interface, we need to know where the price feeds are located. This can be found in the Chainlink Avalanche Feeds documentation. We’re using the address for the AVAX/USD feed, so we can simply initialize the price feed interface with that address as its only parameter when the contract is constructed, like so: `priceFeed = AggregatorV3Interface(0x5498BB86BC934c8D34FDA08E81D444153d0D06aD);`
Once initialized, we can get the latest price data from the aggregator interface by calling its function, `latestRoundData()`, as seen in `getLatestPriceData()`. This returns multiple points of information about the feed, but it’s simply the price we care about, so we return only that. Since the function is not modifying anything and is only reading data from the aggregator interface, it’s defined as a view function, which happily doesn’t use gas.
Compiling and Deploying the Contract
Deploying this code is simple and thanks to Avalanche’s EVM compatibility, there’s very little change required from the standard Ethereum deployment path. Start by compiling in Remix under the Compiler tab; simply click “Compile AvaxLinkFeeds.sol”. Then, proceed to the Deploy tab, set the environment to Injected Web3 (MetaMask), and configure your MetaMask for Avalanche’s Fuji testnet. To do this, simply add these settings into your MetaMask networks as a “Custom RPC”.
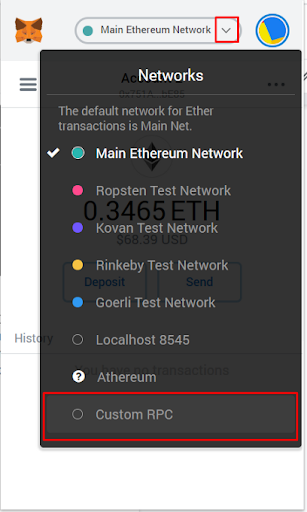
Network Name: Avalanche FUJI C-Chain
New RPC URL: https://api.avax-test.network/ext/bc/C/rpc
ChainID: 43113
Symbol: AVAX
Explorer: https://cchain.explorer.avax-test.network
Then proceed to https://faucet.avax-test.network/ to retrieve some free testnet AVAX for deploying your contract. For more info on this setup process, you can view the Deploy a Smart Contract on Avalanche Documentation.
Now that the contract is compiled, your network is set to Fuji, and your address is funded with testnet AVAX, you can deploy to the network simply by selecting the `AvaxLinkFeeds` contract and clicking Deploy. Your contract is now live on Avalanche and ready to react to real-world data with Chainlink.
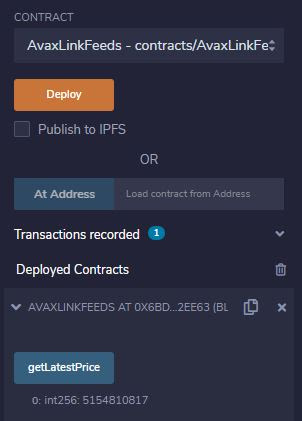
Simply call getLatestPrice and you’ll see AVAX/USD returned with 8 decimals of precision, the value here is $51.54.
That’s it: an import, a constructor that initializes the feed, and a single function to read the price data (without gas!) are all that’s needed to empower your smart contract with Chainlink’s industry-standard price data.
Summary
Avalanche offers a powerful option for building smart contract applications, with the high speed and low cost of the network making it an attractive prospect for developers. When combined with Chainlink decentralized oracle networks, Avalanche becomes more powerful still, able to access off-chain data and events. Chainlink Price Feeds offer high-quality aggregated price data that can be used in all kinds of useful applications, such as decentralized exchanges (DEXs), liquidity pools, lending protocols, decentralized insurance solutions, and automated market makers (AMMs).
If you’d like to learn more about building on Avalanche, watch this workshop where Connor Daly from Ava Labs provides a walkthrough of the process of building your first dApp on Avalanche.
Chainlink is the industry standard for building, accessing, and selling oracle services needed to power hybrid smart contracts on any blockchain. Chainlink oracle networks provide smart contracts with a way to reliably connect to any external API and leverage secure off-chain computations for enabling feature-rich applications. Chainlink currently secures tens of billions of dollars across DeFi, insurance, gaming, and other major industries, and offers global enterprises and leading data providers a universal gateway to all blockchains.
Learn more about Chainlink by visiting chain.link or read the documentation at docs.chain.link. To discuss an integration, reach out to an expert.